
Detecting Touches
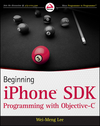
Time to get the engine rolling! Make sure you download the code indicated here so you can work through the following Try It Out activity.
This project [MultiTouch.zip] is available for download at Wrox.com.
Try It Out Detecting for Taps
1. Using Xcode, create a new View-based Application project and name it MultiTouch.
2. Drag and drop an image into the Resources folder. Figure 14-1 shows an image named apple.jpeg located in the Resources folder.

Figure 14-1
3. Double-click the MultiTouchViewController.xib file to edit it in Interface Builder.
4. Populate the View window with the ImageView view. Ensure that the ImageView covers the entire View window.
5. Select the ImageView view and view its Attributes window (see Figure 14-2). Set its Image property to apple.jpeg.

Figure 14-2
6. In the MultiTouchViewController.h file, add the following statements that appear in bold:
#import
@interface MultiTouchViewController : UIViewController {
IBOutlet UIImageView *imageView;
}
@property (nonatomic, retain) UIImageView *imageView;
@end
7. Back in Interface Builder, Control-click and drag the File’s Owner item to the ImageView view. Select ImageView.
8. In the MultiTouchViewController.m file, add the following statements that appear in bold:
#import "MultiTouchViewController.h"
@implementation MultiTouchViewController
@synthesize imageView;
//---fired when the user finger(s) touches the screen---
-(void) touchesBegan: (NSSet *) touches withEvent: (UIEvent *) event {
//---get all touches on the screen---
NSSet *allTouches = [event allTouches];
//---compare the number of touches on the screen---
switch ([allTouches count])
{
//---single touch---
case 1: {
//---get info of the touch---
UITouch *touch = [[allTouches allObjects] objectAtIndex:0];
//---compare the touches---
switch ([touch tapCount])
{
//---single tap---
case 1: {
imageView.contentMode = UIViewContentModeScaleAspectFit;
} break;
//---double tap---
case 2: {
imageView.contentMode = UIViewContentModeCenter;
} break;
}
} break;
}
}
- (void)dealloc {
[imageView release];
[super dealloc];
}
9. Press Command-R to test the application on the iPhone Simulator.
10. Single-tap the apple icon to enlarge it. Double-tap it to return it to its original size (see Figure 14-3).

Figure 14-3
How It Works
The preceding application works by sensing the user’s touch on the screen of the iPhone or iPod Touch. When the user touches the screen, the View or View Controller fires a series of events that you can handle. There are four such events:
- touchesBegan:withEvent:
- touchesEnded:withEvent:
- touchesMoved:withEvent:
- touchesCancelled:withEvent:
Take a closer look at the first event. First, the touchesBegan:withEvent: event is fired when at least one touch is sensed on the screen. In this event, you can know how many fingers are on the screen by calling the allTouches method of the UIEvent object (event):
//---get all touches on the screen---
NSSet *allTouches = [event allTouches];
The allTouches method returns an NSSet object containing a set of UITouch objects. To know how many fingers are on the screen, simply count the number of UITouch objects in the NSSet object using the count method. In this case, you are (at this moment) interested only in a single touch, therefore you implement only the case for one touch:
//---compare the number of touches on the screen---
switch ([allTouches count])
{
//---single touch---
case 1: {
//---get info of the touch---
UITouch *touch = [[allTouches allObjects] objectAtIndex:0];
//---compare the touches---
switch ([touch tapCount])
{
//---single tap---
case 1: {
imageView.contentMode = UIViewContentModeScaleAspectFit;
} break;
//---double tap---
case 2: {
imageView.contentMode = UIViewContentModeCenter;
} break;
}
} break;
}
You extract details of the first touch by using the allObjects method of the NSSet object to return an NSArray object. You then use the objectAtIndex: method to obtain the first array item.
The UITouch object (touch) contains the tapCount property, which tells you whether the user has single-tapped the screen or performed a double tap (or more). If the use single-tapped the screen, you resize the image to fit the entire ImageView view using the UIViewContentModeScaleAspectFit constant. If it is a double-tap, you restore it to its original size using the UIViewContentModeCenter constant.
The other three events, which are not discussed in this section, are touchesEnded:withEvent:, touchesMoved:withEvent:, and touchesCancelled:withEvent:.
The touchesEnded:withEvent: event is fired when the user’s finger(s) is lifted from the screen. The touchesMoved:withEvent: event is fired continuously when the user’s finger or fingers are touching and moving on the screen. Finally, if the application is interrupted while the user’s finger is on the screen, the touchesCancelled:withEvent: event is fired.
NOTE: In addition to detecting taps in the touchesBegan:withEvent: event, you can detect them in the touchesEnded:withEvent: event.
No comments:
Post a Comment